누구나 피아노 작곡을 할 수 있다. 단, AI와 함께라면 ...
피아노로 아름다운 곡을 듣거나 연주할 때면 이걸 직접 작곡할 수 없을까 하는 생각을 해보았을 거다. AI가 못하는 게 없는 세상인데 이 정도는 어렵지 않을 것 같아 작업을 시켜봤는데 결과는 놀라웠다.
작곡에서 가장 중용한 건 화음이다. 기본음을 정해 시작하고 다음 화음을 연결하는 방식이다. 이러한 방식으로 곡이 완성되기 때문에 이것을 AI에게 알려 주기만 하면 나머지는 알아서 만든다.
1. 작곡 규칙 정하기
AI에 작업을 시키기 전에 먼저 작곡 룰을 만들자. 유튜브에서 찾은 간단한 작곡 규칙이다. 시작과 끝 그리고 중간 연결화음 정의가 되어있다. 이거면 충분하다. 본격적으로 작업을 시켜보자.
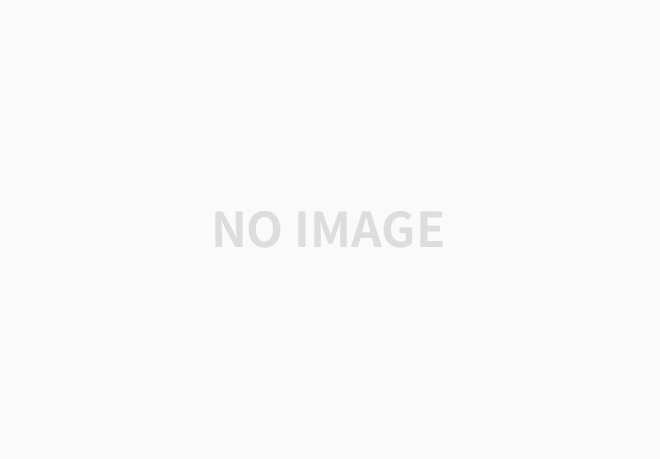
2. 작곡 프롬프트 작성
첨부한 화음규칙에 맞춰 8마디 작곡을 하는 파이썬 코드를 작성해줘. 그리고 악보를 확인할 수 있는 앱과 연동할 수 있도록 해줘.
import random
from typing import List, Tuple
import os
from music21 import *
class MusicComposer:
def __init__(self):
self.harmony_rules = {
'I': ['ii', 'iii', 'IV', 'V', 'vi'],
'ii': ['IV', 'V'],
'iii': ['ii'],
'IV': ['ii', 'V'],
'V': ['I', 'ii'],
'vi': ['ii', 'IV', 'V']
}
self.note_mapping = {
1: 'C4',
2: 'D4',
3: 'E4',
4: 'F4',
5: 'G4',
6: 'A4',
7: 'B4'
}
self.chord_notes = {
'I': [1, 3, 5],
'ii': [2, 4, 6],
'iii': [3, 5, 7],
'IV': [4, 6, 1],
'V': [5, 7, 2],
'vi': [6, 1, 3]
}
# 리듬 패턴 정의 (각 패턴의 합은 4.0이어야 함)
self.rhythm_patterns = [
[1.0, 1.0, 1.0, 1.0], # 4분음표 4개
[1.0, 0.5, 0.5, 1.0, 1.0], # 4분음표-8분음표2개-4분음표2개
[1.0, 1.0, 0.5, 0.5, 1.0], # 4분음표2개-8분음표2개-4분음표
[0.5, 0.5, 1.0, 1.0, 1.0], # 8분음표2개-4분음표3개
]
def generate_chord_progression(self) -> List[str]:
progression = ['I']
for _ in range(6):
current = progression[-1]
next_chord = random.choice(self.harmony_rules[current])
progression.append(next_chord)
if progression[-1] != 'V':
progression[-1] = 'V'
progression.append('I')
return progression
def generate_melody(self, chord_progression: List[str]) -> List[tuple[List[int], List[float]]]:
"""멜로디와 리듬을 생성"""
melody = []
for chord in chord_progression:
available_notes = self.chord_notes[chord]
# 랜덤하게 리듬 패턴 선택
rhythm = random.choice(self.rhythm_patterns)
# 리듬 패턴 길이만큼 음표 생성
notes = [random.choice(available_notes) for _ in range(len(rhythm))]
melody.append((notes, rhythm))
return melody
def create_score(self, chord_progression: List[str], melody: List[tuple[List[int], List[float]]],
filename: str = "composition") -> str:
score = stream.Score()
# 멜로디 파트
melody_part = stream.Part()
melody_part.append(instrument.Piano())
melody_part.append(meter.TimeSignature('4/4'))
melody_part.append(key.Key('C'))
# 반주 파트
accompaniment_part = stream.Part()
accompaniment_part.append(instrument.Piano())
accompaniment_part.append(meter.TimeSignature('4/4'))
accompaniment_part.append(key.Key('C'))
# 멜로디 노트 추가
for bar_idx, (bar_notes, bar_rhythm) in enumerate(melody):
measure = stream.Measure(number=bar_idx + 1)
for note_num, note_length in zip(bar_notes, bar_rhythm):
note_name = self.note_mapping[note_num]
n = note.Note(note_name, quarterLength=note_length)
measure.append(n)
melody_part.append(measure)
# 반주 (2분음표 코드) 추가
for chord_idx, chord_name in enumerate(chord_progression):
measure = stream.Measure(number=chord_idx + 1)
chord_notes = self.chord_notes[chord_name]
chord_note_names = [self.note_mapping[n] for n in chord_notes]
# 한 마디에 2분음표 코드 2개
for _ in range(2):
c = chord.Chord(chord_note_names, quarterLength=2.0)
measure.append(c)
accompaniment_part.append(measure)
score.append(melody_part)
score.append(accompaniment_part)
xml_path = f"{filename}.musicxml"
score.write('musicxml', xml_path)
return xml_path
def compose_and_visualize(self):
chord_progression = self.generate_chord_progression()
melody = self.generate_melody(chord_progression)
print("화음 진행:", ' '.join(chord_progression))
print("\n멜로디 (음표, 리듬):")
for bar_num, ((notes, rhythm), chord) in enumerate(zip(melody, chord_progression), 1):
print(f"마디 {bar_num} ({chord}): 음표={notes}, 리듬={rhythm}")
xml_file = self.create_score(chord_progression, melody)
print(f"\nMusicXML 파일이 생성되었습니다: {xml_file}")
try:
if os.name == 'nt':
os.startfile(xml_file)
else:
os.system(f'open {xml_file}')
except Exception as e:
print("악보를 자동으로 열 수 없습니다. MuseScore에서 직접 파일을 열어주세요.")
def main():
try:
import music21
except ImportError:
print("필요한 패키지를 설치합니다...")
os.system("pip install music21")
print("패키지 설치가 완료되었습니다. 프로그램을 다시 실행해주세요.")
return
composer = MusicComposer()
composer.compose_and_visualize()
if __name__ == "__main__":
main()
3. MuseScore 앱 설치 (https://musescore.com/)
AI가 작곡한 악보와 음악을 듣기 위해 MusesScore를 설치한다. 무료임. 파이썬코드를 실행하면 앱 연동하여 자동 실행된다. MusesScore에서 악보 수정도 편하게 할 수 있다.
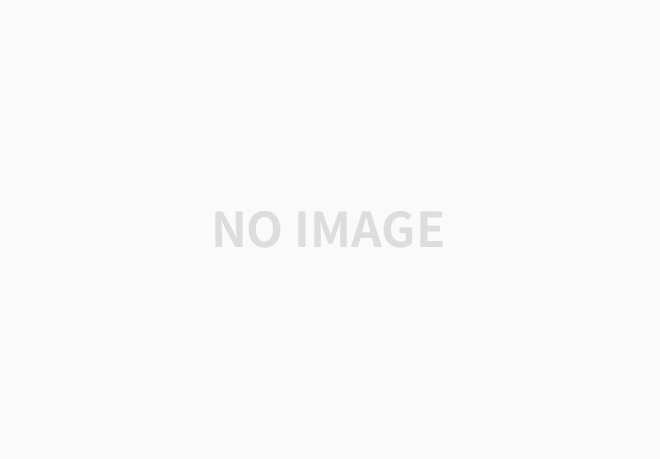
4. 작곡 실행 영상
8마디 간단한 피아노 연습곡이 완성되었다. 화음에 맞는 멜로디를 구성하게 했다. 단순해 보이지만 화음 규칙을 맞췄기 때문에 다음 마디가 자연스럽게 연결된다. 8분 음표와 4분 음표 외 다양한 음표와 리듬을 추가해 작곡을 하면 훌륭한 연주가 될 수도 있을 것이다.
작곡이야 말로 어쩌면 AI가 가장 쉽게 할 수 있는 분야가 아닐까하는 생각이 든다. 왜냐하면 다음 코드르 보면 이해가 바로 될 것이다. 고전 천재적인 음악가들 모차르트나 쇼팽 같은 대가들이 어떤 식으로 작곡을 했었는지는 모르지만, 요즘 대부분의 작곡은 이러한 어느 정도 정해진 규칙을 따라 곡을 만들 것 같다. 그렇다면 그 룰들을 수집해 AI에 랜덤하게 생성하게 하면 멋진 곡이 나올 확률이 높아지지 않겠는가... :)
하모니 룰이다.
코드 구성은 다음과 같다.
심지어 패턴은 다음과 같이 정의한다 .
'코드리뷰 > chatGPT(Python)코드' 카테고리의 다른 글
AI 성수동 케이크 맛집 검색 사이트 만들기 (1) | 2025.02.07 |
---|---|
AI 인공지능 프리젠테이션 만들기 자동화 (0) | 2025.02.07 |
광화문 맛집 찾기 10분 만에 만들기 - DeepSeek, Cluade, ChatGpt 이용 (3) | 2025.02.02 |
댓글